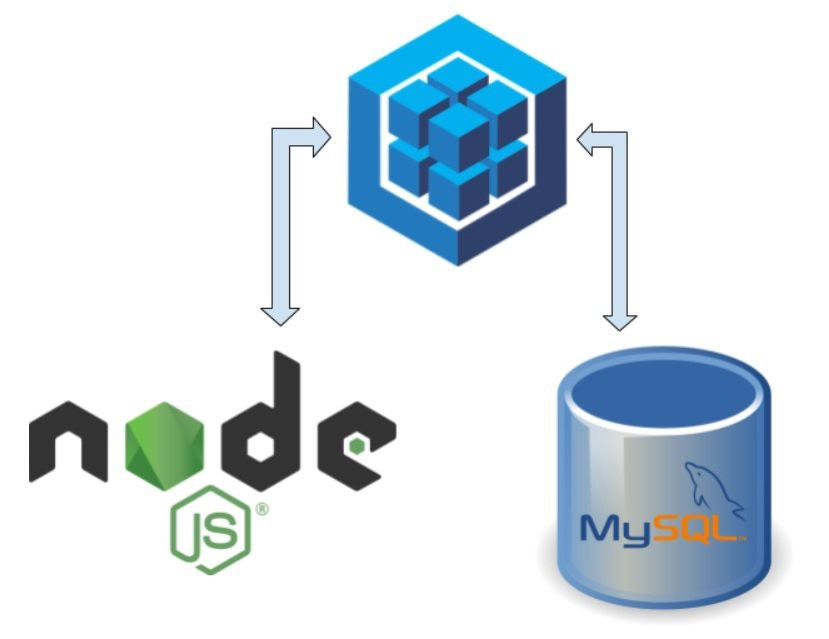
This article will helps to develop a basic API through Node.js, ExpressJS and Sequelize ORM with PostgreSQL/MySQL.
For this project we will use below packages and software:
Node.js : JS Runtime environment
ExpressJS : Web framework
Sequelize and Sequelize-cli: ORM, click here to see docs
Cors: Cross Origin
Nodemon: Helps tp develop Node.js application by automatically restarting the node application.
PostgreSQL: DB to perform CRUD.
Nodejs can be installed though this link here
Once you have installed the Nodejs in your system, you need to create a project directory.
I have created crud-api, go to your project directory through command prompt and type below command
npm init -y
This command will create a package.json file, node_modules folder.
Now we need to install few packages to make things work for this project. These packages are Postgres, nodemon, cors, express etc.
npm install --save express sequelize sequelize-cli nodemon pg cors
Create one file in your directory app.js.
After that type below command to initialize sequelize orm.
npx sequelize init
This command will create config, migrations, seeders, models folders inside your directory.
So far we have created a skeleton of our project. Below things are yet to create.
Changes in config.js file: change your DB name, user, password in config.js which is in config folder. Make sure do these changes in development object.
"development": {
"username": "YOUR_DB_USER_NAME",
"password": "YOUR_DB_PASSWORD",
"database": "YOUR_DB_NAME",
"host": "127.0.0.1",
"dialect": "pg"
}
Once you've done with the changes, type below code in app.js.
var express = require('express');
var cors = require('cors');
var app = express();
app.use(cors());
app.use(express.json());
app.get('/', function(req, res) {
res.send('working')
});
app.listen(4000, () => {
console.log('this is running')
});
type this command "npx nodemon app.js" and test in your browser with localhost:4000/ url.
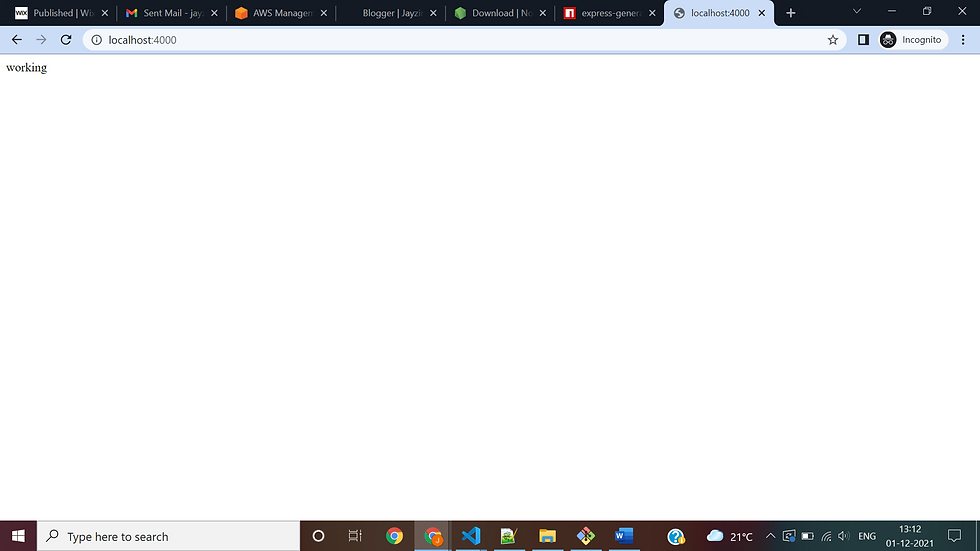
Now we have to create one model, migration, seeders for DB operations.
Type below command to create Model with migration.
npx sequelize-cli model:generate --name Users --attributes name:string,email:string
You will one file in models folder and one file in migrations folder.
If you want to change your schema, you can add or remove column name from migrations file. Once done type below command to run the migration and create users table.
npx sequelize-cli db:migrate
Now you will get your table in DB.
Seed some data inside it. For seed, create a new seed file with the below command
npx sequelize-cli seed:generate --name users-seed
paste below code in seed file you have created 'users-seed.js' in seeders folder.
'use strict';
module.exports = {
up: async (queryInterface, Sequelize) => {
await queryInterface.bulkInsert('Users', [
{
name: "John",
email: "john.doe@email.com",
createdAt: new Date(),
updatedAt: new Date()
},
{
name: "Morgan",
email: "morgan@email.com",
createdAt: new Date(),
updatedAt: new Date()
}
])
},
down: async (queryInterface, Sequelize) => {
/**
* Add commands to revert seed here.
*
* Example:
* await queryInterface.bulkDelete('People', null, {});
*/
}
};
Type this command and seed your data in users table
npx sequelize-cli db:seed:all
Now we have create DB, Table with data. Its time to create CRUD API
Include the below code in app.js file
var Models = require('./models');
var express = require('express');
var cors = require('cors');
var app = express();
app.use(cors());
app.use(express.json());
// to read data
app.get('/read', async function(req, res) {
let list = await Models.Users.findAll();
res.send({
status: 200
data: list
});
});
//to delete
app.post('/delete', async function(req, res) {
let {userId} = req.body;
if (!userId) {
return res.status(401).send({message: "Unauthorized"});
}
await Models.Users.destroy({
where: { id: userId }
});
return res.status(200).send({message: "Data deleted successfully"});
});
//to update
app.post('/delete', async function(req, res) {
let {userId, name, email} = req.body;
if (!userId) {
return res.status(401).send({message: "Unauthorized"});
}
await Models.Users.update({
name:name,
email:email
},{
where: { id: userId }
}).catch(err => res.status(401).send({message: "record not updated"}));
return res.status(200).send({message: "record updated successfully"});
});
//create
app.post('/create', async function(req, res) {
let {name, email} = req.body;
await Models.Users.create({
}).catch(error => res.status(200).send({message: "unable to create"}));
return res.status(200).send({message: "record created successfully"});
});
//port 4000
//url http://localhost:400/
app.listen(4000, () => { console.log('this is working'); });
//you can test the output through postman and get the result
//http://localhost:4000/read
//METHOD: GET
//http://localhost:4000/create
//METHOD: POST
// {
// "name" : "Mohan",
// "email" : "mohan@mail.com"
// }
//http://localhost:4000/update
//METHOD: POST
// {
// "userId": 1212,
// "name": "mini",
// "email": "mini@mail.com"
// }
//http://localhost:4000/delete
//METHOD: POST
// {
// "userId": 1212
// }
Comments